How to build a table of contents for Sanity in a Next.js website
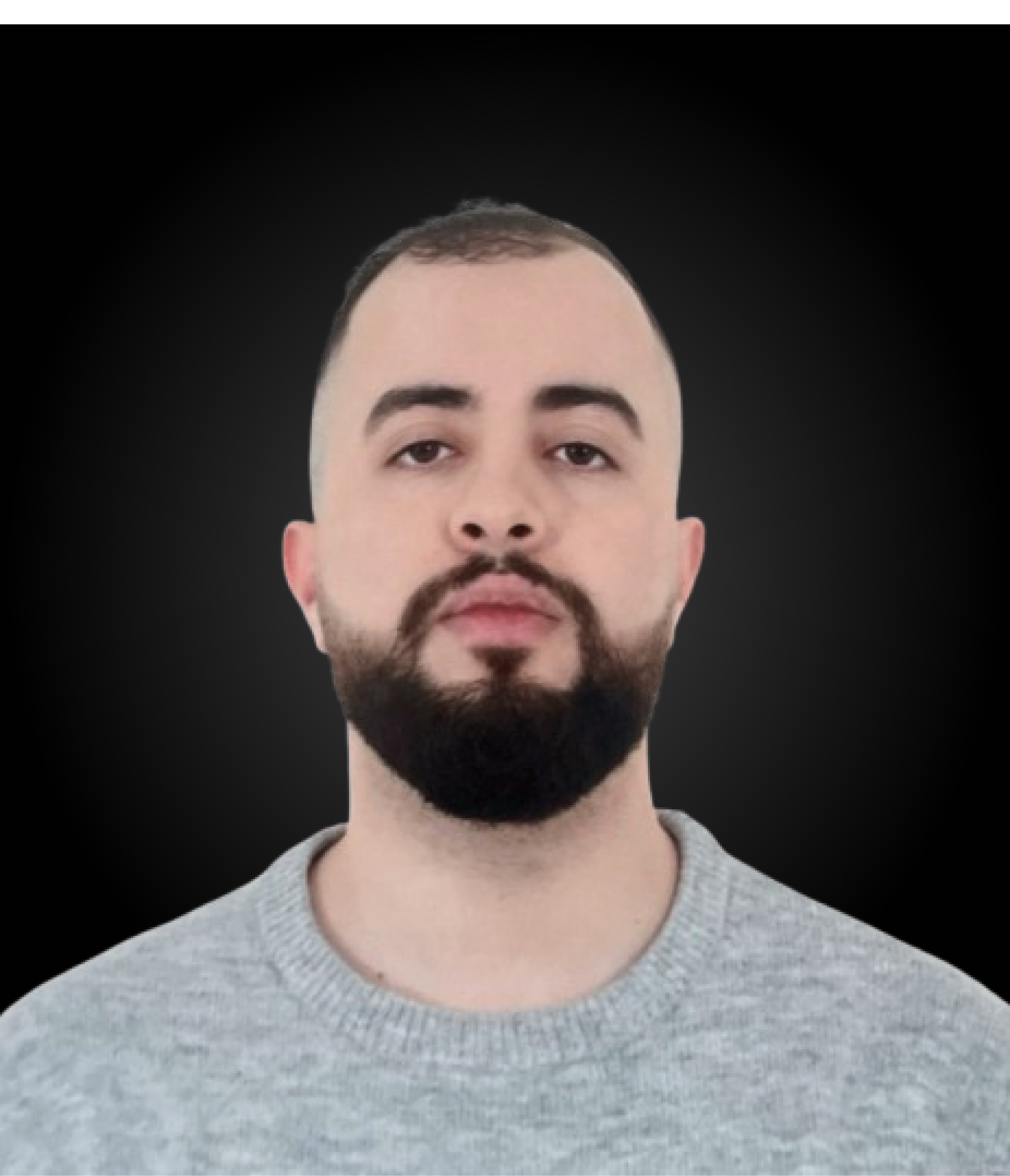
Imad Attif, Sr. Frontend Engineer
4 min read
Feb 5, 2025
In this tutorial, I'll show you how to build a Table of Contents (ToC) for a Next.js website that integrates with Sanity. This ToC will automatically extract headings from your content and allow users to navigate your page easily. I'll walk you through the process step-by-step, explaining how each part of the code works, and how you can customize it for your website.
Prerequisites
Before we start, ensure you have the following:
- A Next.js project set up.
- A Sanity project with Portable Text content (for structured content).
- Since we will be using Tailwind CSS to style the Table of Contents, you need to have Tailwind CSS installed in your Next.js project.
- Basic knowledge of React and Sanity.
What You’ll Learn
By the end of this tutorial, you will know how to:
- Extract headings (like
h2
) from Portable Text in Sanity. - Track and highlight the current heading as users scroll through the page.
- Implement both a dropdown and a list variant for the ToC.
- Enable smooth scrolling to specific sections when a heading is clicked.
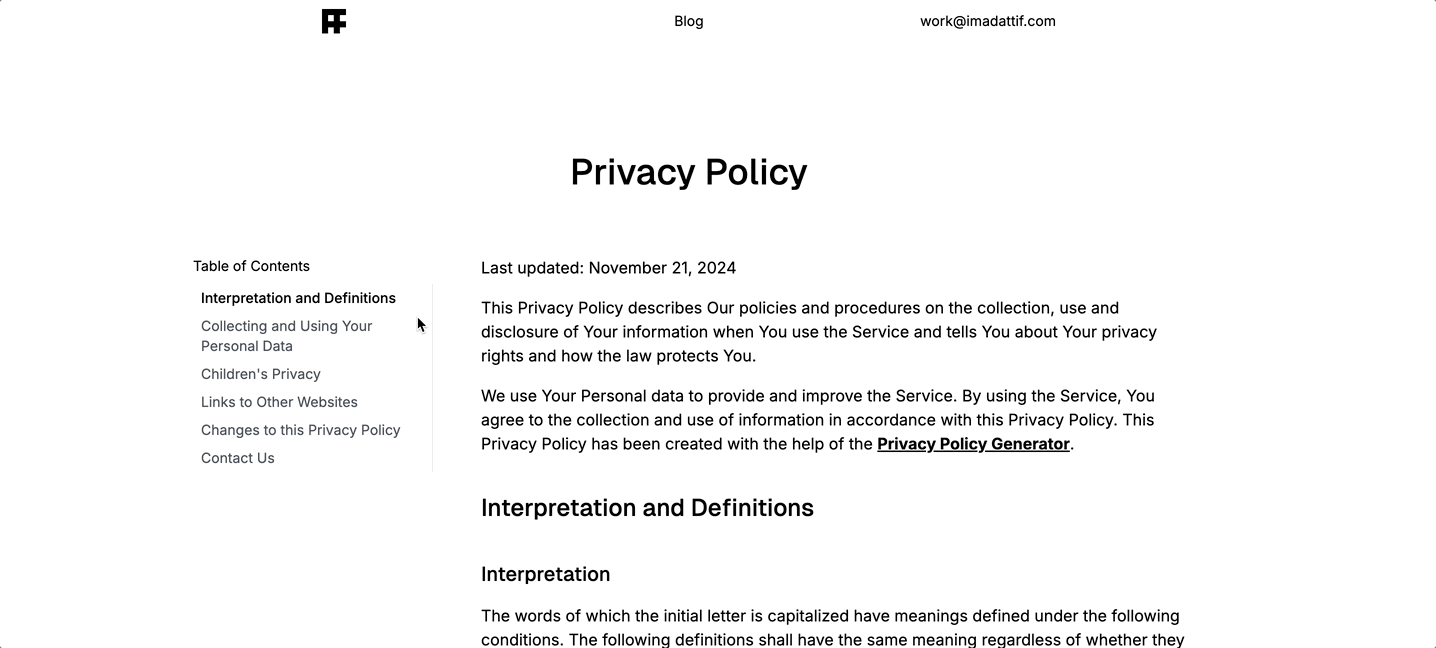
Create the TableOfContents Component
In this step, we will build the core component for the Table of Contents. This component will extract headings from your content and allow users to click on them to scroll to the respective sections.
Define Component Props
We define the TableOfContents props so we can customize the component's behavior.
table-of-contents.tsx
- className: Custom CSS class for styling.
- title: An optional title for the Table of Contents.
- variant: Whether to display the ToC as a list (default) or dropdown (dropdown).
- value: The content passed to the component, typically a Portable Text object from Sanity.
Extract Headings from Portable Text
We need a function that will extract the h2
headings from the Portable Text content. Let’s create a utility to get these headings.
helpers.ts
- getHeadingText: Extracts the text of each heading by joining the children array.
- getContentHeadings: Loops through the Portable Text nodes, extracts
h2
nodes, and returns an array with their id and text content.
This process converts the Portable Text into a list of headings:
example.json
Track Scroll Position
We'll use React's useState
and useEffect
to track the current heading in view when users scroll the page. This ensures the active heading is highlighted.
table-of-contents.tsx
handleScroll: This function checks the position of each heading in the viewport and updates the currentHeading when the heading is in view.
Handle Heading Click for Smooth Scrolling
To enable smooth scrolling when a heading is clicked, we define a function that scrolls the page to the corresponding section.
table-of-contents.tsx
This function:
- Prevents the default anchor behavior.
- Scrolls smoothly to the selected heading with an offset.
- If in dropdown mode, closes the dropdown and sets the selected heading text.
Render Table of Contents
Next, we will render the headings dynamically. Depending on the variant
prop, the ToC can either display a list or a dropdown.
Default List
The default ToC renders a simple list of links. When a heading is clicked, it smoothly scrolls to the corresponding section.
table-of-contents.tsx
Dropdown Variant
For the dropdown variant, we display the headings in a dropdown list that is toggled by clicking a button.
table-of-contents.tsx
The dropdown renders the list inside a collapsible menu that is shown when the button is clicked.
Fetch Sanity Content and Integrate the ToC Component
Now, let’s fetch content from Sanity and pass it to the TableOfContents
component.
Article
Conclusion
By following this tutorial, you've learned how to build a Table of Contents for your Next.js website using Sanity. This ToC:
- Extracts h2 headings from Portable Text content.
- Tracks and highlights the currently visible heading as users scroll.
- Offers two display variants: a simple list and a dropdown.
- Enables smooth scrolling to specific sections when a heading is clicked.
You can further enhance this ToC by adding more customization options, such as supporting multiple heading levels or adding animations!